I think the reply will depend on what kind of arrangement you want to use. (e.g. I want to rotate the object).
For example,
here is a script.
The way to do it is to apply weight paint to the part of the mesh you want to place.
Only the empties in the specified collection will move with the distribution of the weight paint on the mesh.
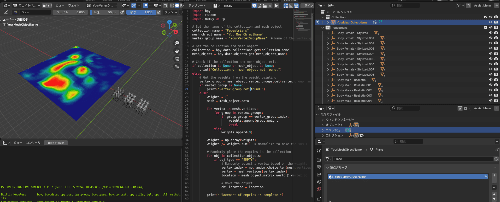
import bpy
import random
import numpy as np
# Set the names of the collection and mesh object
collection_name = "Pedestrians"
mesh_object_name = "YourMeshObjectName"
vertex_group_name = "YourVertexGroupName" # Name of the vertex group with weight painting
# Get the collection and mesh object
collection = bpy.data.collections.get(collection_name)
mesh_object = bpy.data.objects.get(mesh_object_name)
# Check if the collection and mesh object exist
if collection is None or mesh_object is None:
print("Collection or mesh object not found.")
else:
# Get the weights from the weight painting
vertex_group = mesh_object.vertex_groups.get(vertex_group_name)
if vertex_group is None:
print("Vertex group not found.")
else:
weights = []
mesh = mesh_object.data
for vertex in mesh.vertices:
for group in vertex.groups:
if group.group == vertex_group.index:
weights.append(group.weight)
break
else:
weights.append(0)
weights = np.array(weights)
weights /= weights.sum() # Normalize to make the sum 1
# Randomly place the empties in the collection
for obj in collection.objects:
if obj.type == 'EMPTY':
# Randomly select a vertex based on the weights
vertex_index = np.random.choice(len(mesh.vertices), p=weights)
vertex = mesh.vertices[vertex_index]
location = mesh_object.matrix_world @ vertex.co # Convert to world coordinates
# Move the object
obj.location = location
print("Placement of empties is complete.")
Supplementation
if the person is the same mesh, it is better to make an instance collection.
If the person is the same mesh, it would be more memory efficient to make instance collections
memory efficiency.