You could search through all blends with a python script. I suppose your clue to the object is its name. The following method finds all objects with a given name and returns their file locations.
Go to the top level directory, from which you want to search files in all subdirectories. (If you're crazy enough, this might just be the root of your drive.)
Create a new file name search_blend.py.
Open it and enter the following script.
import bpy
import os
import os.path
name = "Cube"
save_file = "D:/blndtmp/results.txt"
objects = bpy.data.objects
for o in objects:
if o.name == name:
if os.path.exists(save_file):
append_write = 'a' # append if already exists
else:
append_write = 'w'
with open(save_file, append_write) as save_file:
save_file.write("Found " + name + " in " + bpy.data.filepath + ".\n")
This script will be executed by every blend file, which we find. You will have to change the name
variable to your objects name. name = "my objects name"
Furthermore you will have to set the path in which we save the results. I save my results to my path D:/blndtmp/results.txt
. The following lines loop through all objects, compare their name with the search name and write to the save_file if an object with the same name is found.
Assuming you have no python installed, lets use Blenders internal python installation. Open up Blender and save the file in the same directory as the search_blend.py script. I named my file empty_blend.
Your folder will now include these files.
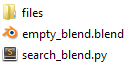
In the empty_blend open up a text editor and paste this script inside.
import os
import subprocess
blender_path = "C:/Program Files/Blender Foundation/Blender/blender.exe"
searched_files = []
for root,dirs,files in os.walk(os.getcwd()):
for f in files:
if f[-6:] == ".blend":
path = os.path.join(root,f)[len(os.getcwd()) + 1:]
searched_files.append(path)
subprocess.call([blender_path, path, "--background","--python", "search_blend.py"])
print(searched_files)
Correct the blender_path variable to your Blender path. You are now ready to execute the script. You may want to open up the Console first to see if it is running correctly. The script searches for all files in all subdirectories with os.walk()
, compares their extension, and if it is a .blend file, executes is in background mode deploying the search_blend.py script.
Hit Run Script in the text editor to run the script.
After its completion, it will have created a new file next to search_blend.py and empty_blend.blend, if an object was found. It is the results path, which we entered in the search_blend.py script.
My results.txt looked like this after a test run.
Found Cube in D:\blndtmp\files\a.blend.
Found Cube in D:\blndtmp\files\c.blend.
Found Cube in D:\blndtmp\files\more files\a.blend.
Found Cube in D:\blndtmp\files\more files\c.blend.