In the glTF export dialog, untick Data > Mesh > UVs and you get your 8 vertices and normals. The other option is to remove the automatically created UVMap under the object's Data > UV Maps panel (then Data > Mesh > UVs can stay ticked).
Edit:
Why does the glTF file for the default cube has 14 vertices?
Open Blender, set the shading of the default cube to Shade Smooth and run this script:
import bpy
obj = bpy.data.objects["Cube"]
# print all vertices with their uvs defining the cube
pairs = []
for face in obj.data.polygons:
for vert_index, loop_index in zip(face.vertices, face.loop_indices):
uv_coords = obj.data.uv_layers.active.data[loop_index].uv
pairs.append((face.index, vert_index, uv_coords.x, uv_coords.y))
print("face: %i, vertex: %i, uvs: %f, %f" % pairs[-1])
# print all vertices with their uvs which are unique
# and therefore must be stored
uniques = []
for pair in pairs:
found = False
for other in uniques:
if pair[1] == other[1] and pair[2] == other[2] and pair[3] == other[3]:
found = True
break
if not found:
uniques.append(pair)
print(len(uniques), uniques)
This gives (Window > Toggle System Console):
face: 0, vertex: 0, uvs: 0.625000, 0.500000
face: 0, vertex: 4, uvs: 0.875000, 0.500000
face: 0, vertex: 6, uvs: 0.875000, 0.750000
face: 0, vertex: 2, uvs: 0.625000, 0.750000
face: 1, vertex: 3, uvs: 0.375000, 0.750000
face: 1, vertex: 2, uvs: 0.625000, 0.750000
face: 1, vertex: 6, uvs: 0.625000, 1.000000
face: 1, vertex: 7, uvs: 0.375000, 1.000000
face: 2, vertex: 7, uvs: 0.375000, 0.000000
face: 2, vertex: 6, uvs: 0.625000, 0.000000
face: 2, vertex: 4, uvs: 0.625000, 0.250000
face: 2, vertex: 5, uvs: 0.375000, 0.250000
face: 3, vertex: 5, uvs: 0.125000, 0.500000
face: 3, vertex: 1, uvs: 0.375000, 0.500000
face: 3, vertex: 3, uvs: 0.375000, 0.750000
face: 3, vertex: 7, uvs: 0.125000, 0.750000
face: 4, vertex: 1, uvs: 0.375000, 0.500000
face: 4, vertex: 0, uvs: 0.625000, 0.500000
face: 4, vertex: 2, uvs: 0.625000, 0.750000
face: 4, vertex: 3, uvs: 0.375000, 0.750000
face: 5, vertex: 5, uvs: 0.375000, 0.250000
face: 5, vertex: 4, uvs: 0.625000, 0.250000
face: 5, vertex: 0, uvs: 0.625000, 0.500000
face: 5, vertex: 1, uvs: 0.375000, 0.500000
14 [(0, 0, 0.625, 0.5), (0, 4, 0.875, 0.5), (0, 6, 0.875, 0.75), (0, 2, 0.625, 0.75), (1, 3, 0.375, 0.75), (1, 6, 0.625, 1.0), (1, 7, 0.375, 1.0), (2, 7, 0.375, 0.0), (2, 6, 0.625, 0.0), (2, 4, 0.625, 0.25), (2, 5, 0.375, 0.25), (3, 5, 0.125, 0.5), (3, 1, 0.375, 0.5), (3, 7, 0.125, 0.75)]
As one can see, there are 14 unique uv'ed vertices to keep track of.
Edit2: note that there are only 8 vertices as it should be. A vertex (representing only a position) is not the same as an uv'ed vertex (representing a position and an uv attribute).
Do the same with an extruded plane, this will give this output:
face: 0, vertex: 0, uvs: 0.000000, 0.000000
face: 0, vertex: 2, uvs: 0.000000, 1.000000
face: 0, vertex: 3, uvs: 1.000000, 1.000000
face: 0, vertex: 1, uvs: 1.000000, 0.000000
face: 1, vertex: 5, uvs: 0.000000, 0.000000
face: 1, vertex: 6, uvs: 1.000000, 0.000000
face: 1, vertex: 7, uvs: 1.000000, 1.000000
face: 1, vertex: 4, uvs: 0.000000, 1.000000
face: 2, vertex: 3, uvs: 1.000000, 1.000000
face: 2, vertex: 2, uvs: 0.000000, 1.000000
face: 2, vertex: 4, uvs: 0.000000, 1.000000
face: 2, vertex: 7, uvs: 1.000000, 1.000000
face: 3, vertex: 0, uvs: 0.000000, 0.000000
face: 3, vertex: 1, uvs: 1.000000, 0.000000
face: 3, vertex: 6, uvs: 1.000000, 0.000000
face: 3, vertex: 5, uvs: 0.000000, 0.000000
face: 4, vertex: 1, uvs: 1.000000, 0.000000
face: 4, vertex: 3, uvs: 1.000000, 1.000000
face: 4, vertex: 7, uvs: 1.000000, 1.000000
face: 4, vertex: 6, uvs: 1.000000, 0.000000
face: 5, vertex: 2, uvs: 0.000000, 1.000000
face: 5, vertex: 0, uvs: 0.000000, 0.000000
face: 5, vertex: 5, uvs: 0.000000, 0.000000
face: 5, vertex: 4, uvs: 0.000000, 1.000000
8 [(0, 0, 0.0, 0.0), (0, 2, 0.0, 1.0), (0, 3, 1.0, 1.0), (0, 1, 1.0, 0.0), (1, 5, 0.0, 0.0), (1, 6, 1.0, 0.0), (1, 7, 1.0, 1.0), (1, 4, 0.0, 1.0)]
Now there are only 8 unique uv'ed vertices, because the created uv map is different. As image (for the default cube, Blender created a nice cube net, but for the extruded plane all sides have the same uv square):
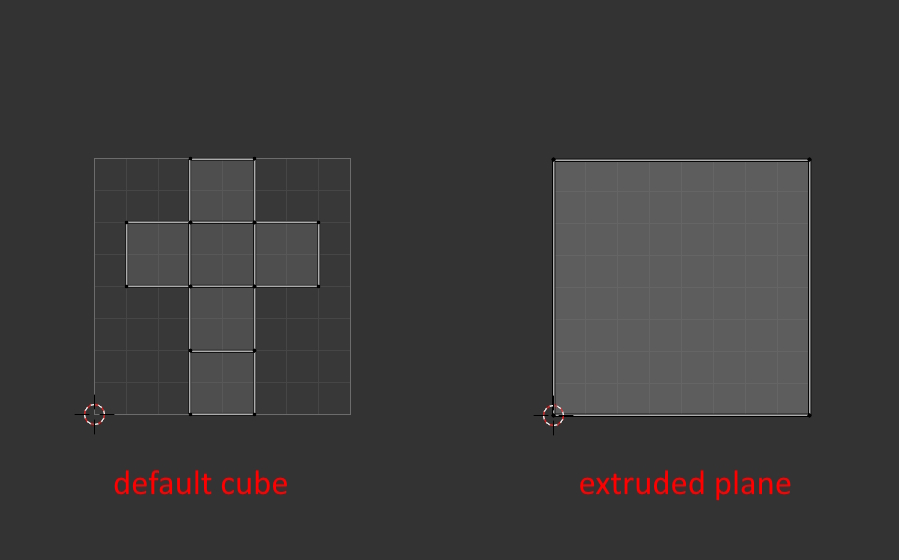
Therefore, the following export glTF behaviour can be observed:
Shade Smooth | Default Cube | Extruded Plane
----------------------------------------------------------
Data > Mesh > UVs ticked | 14 | 8
Data > Mesh > UVs unticked | 8 | 8
In the glTF file the information can be found under:
{
...
"accessors":[
{
"bufferView":0,
...
"count":14, <-- here
...
},
...
}
Note: the other answer states
To handle this there are "duplicate" glTF verts (that have the same position, but different normals). The same thing happens for all other "vertex per poly" data, like UVs.
"Duplicate" verts are inserted only when necessary, so turn off export of anything you don't need—normals, tangents, UVs, vertex colors, materials—to get a smaller glTF vert count.
which is why this question is considered a duplicate.