You can loop over every vertex after initializing different arrays for each of your vertex color layers. You can parse the init data from a file (eg .csv) with python but this is not covered in this answer.
import bpy
import bmesh
obj = bpy.context.selected_objects[0] # Get the currently selected object
vertex_colors = obj.data.vertex_colors
while vertex_colors:
vertex_colors.remove(vertex_colors[0]) # Remove all vertex color layers form the mesh data
bm = bmesh.new() # Create new bmesh object
bm.from_mesh(obj.data) # Init the bmesh with the current mesh
verts_count = len(bm.verts) # Get the number of vertices in the mesh
# Not obligatory, I just use this to populate the vertex color layers)
# This dictionary will map each vertex color layer name to the data for each vertex
data_layers = {
'Temperature': # vertex color layer name 1
[
(1, 0, 0, 1), # Color of vertex index 1
(0, 1, 0, 1), # Color of vertex index 2
(0, 0, 1, 1), # Color of vertex index 3
(1, 1, 1, 1), # Color of vertex index 4...
],
'Velocity': # vertex color layer name 2
[(i / verts_count, i / verts_count, i / verts_count, 1) for i in range(verts_count)],
'Salinity': # vertex color layer name 3
[(i / verts_count, 1 - (i / verts_count), 0.5, 1) for i in range(verts_count)],
}
color_layers = {}
for layer_name in data_layers:
# Create the vertex color layers and store them in a dictionary for easy access by name
color_layers[layer_name] = bm.loops.layers.color.new(layer_name)
for layer_name, layer in color_layers.items():
# Loop over each vertex color layer
for v in bm.verts:
# Loop over every vertex
for loop in v.link_loops:
# Loop over every loop of this vertex (the corners of the faces in which this vertex exists)
try:
loop[layer] = data_layers[layer_name][v.index]
# Get the value from the init dictionary
except IndexError:
loop[layer] = (1, 0, 1, 1)
# Set a placeholder (magenta) value if the mapping array is not long enough
bm.to_mesh(obj.data) # Give the data back to the actual mesh
Then to acces the vertex colors in your material, use the vertex color node :
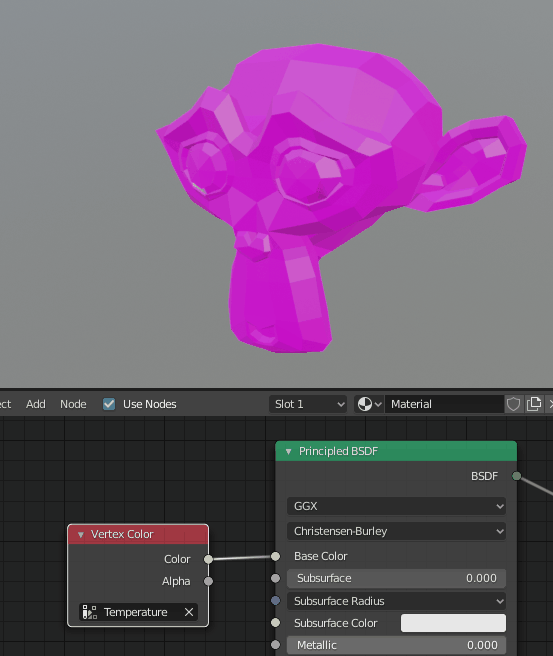
Note that the "Temperature" layer was initialized with only 4 values, so only a few vertices in the right eye got colored and the rest of the vertices got mapped to a magenta color.